PUMPKIN MAN NFT
A randomly generated character project.
Substance Painter / Blender / Cycles / Marvelous Designer / Python
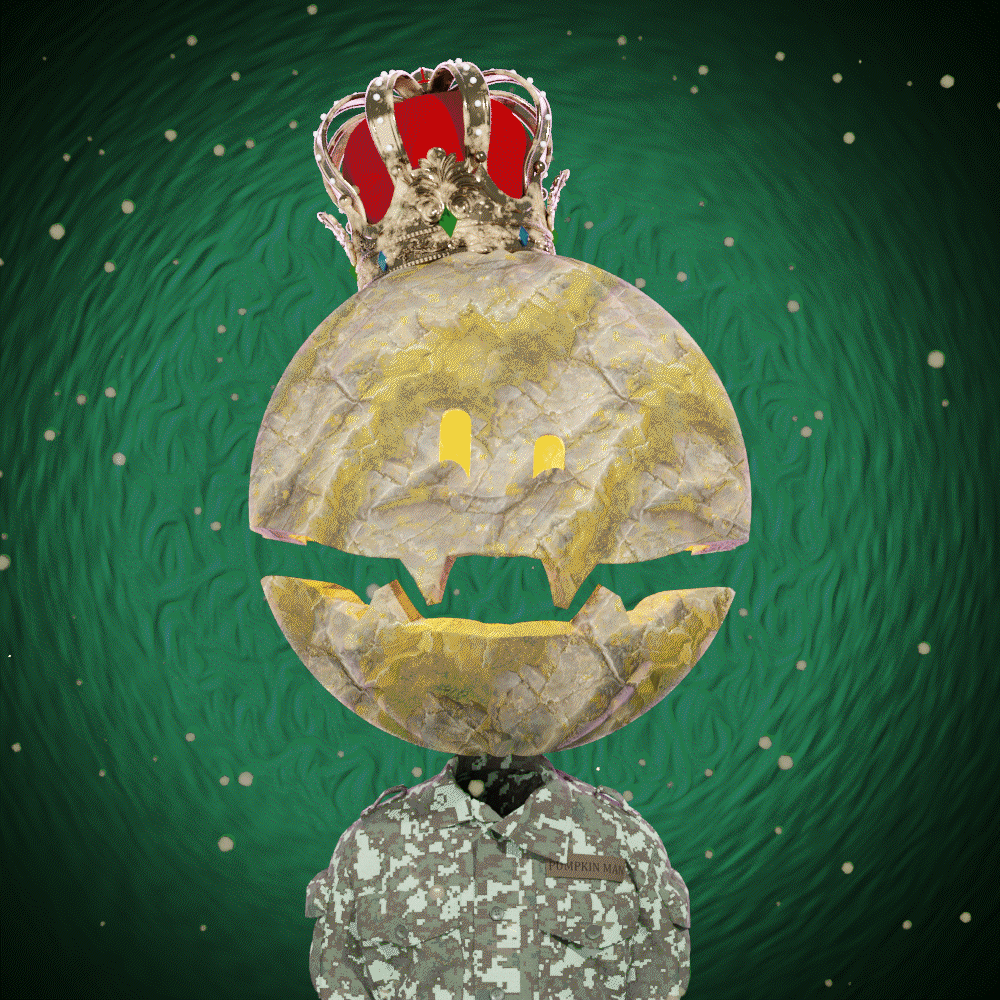

PYTHON CODE
Python in Blender --------------------------
import bpy
import random
from random import randrange
#FUNCTION01 - RANDOM PICK AN OBJECT
def randobj(objname, listname):
#put the objects into the list
for obj in bpy.context.scene.objects:
if obj.name.startswith(objname):
listname.append(obj)
#hide all the objects in the list
for obj in listname:
obj.hide_render = True
#randomly show an object in the list
random.choice(listname).hide_render = False
#FUNCTION02 - RANDOM PICK A MODIFIER
def randmod(objname, modname, listname):
#put the modifiers into the list
for boo in bpy.data.objects[objname].modifiers:
if boo.name.startswith(modname):
listname.append(boo)
#hide all the modifiers in the list
for mod in listname:
mod.show_render = False
#randomly show a modifier in the list
random.choice(listname).show_render = True
#FUNCTION03 - RANDOM PICK A MATERIAL AND ASSIGN IT TO AN OBJECT
def randmat(objname, matname, listname):
#put the materials into the list
for mat in bpy.data.materials:
if mat.name.startswith(matname):
listname.append(mat)
#randomly assign a material to the object
bpy.data.objects[objname].data.materials[0]= random.choice(listname)
#FUNCTION04 - SHOW/HIDE HIERARCHY OBJ
def showhierachy():
for obj in bpy.context.scene.objects:
if obj.hide_render == False:
children = list(obj.children)
for child in children:
child.hide_render = False
if obj.hide_render == True:
children = list(obj.children)
for child in children:
child.hide_render = True
#FUNCTION05 - IF OBJECTS/MODIFIERS SHOW IN RENDER, SHOW THEM IN THE VIEWPORT
def ShowInViewport():
for obj in bpy.context.scene.objects:
obj.hide_viewport = True
obj.hide_set(True)
if obj.hide_render == False:
obj.hide_viewport = False
obj.hide_set(False)
for mod in obj.modifiers:
mod.show_viewport = False
if mod.show_render == True:
mod.show_viewport = True
#FUNCTION06 - RARITY IS THE PROBABILITY OF GENERATING AN ACCESSORY
#rarity = 0 equals to 100%, higher value lower probability
def generate(rarity=0):
bodies = []
hats = []
mouthsBoo = []
eyesBoo = []
eyewears = []
materials = []
accessories = []
#randomly pick a body
randobj("Body", bodies)
#randomly pick a hat
randobj("Hat", hats)
#decrease and customize the chance to generate accessories
for x in range(rarity*10):
accessories.append(bpy.data.objects["null"])
#randomly pick an accessory
randobj("Accessory", accessories)
#balance the probibilty to generate between eyes and eyewears
for x in range(len(eyesBoo)):
eyewears.append(bpy.data.objects["null"])
#randomly pick an eyewear
randobj("Eyewear", eyewears)
#randomly pick a mouth boolean
randmod("Head-002","Mouth",mouthsBoo)
#randomly pick a eyes boolean
randmod("Head-002","Eyes",eyesBoo)
#randomly assign a material to Head
randmat("Head-002", "Head", materials)
#random rotate particles #6 is close to 360 degrees
bpy.data.objects["particle_mesh"].rotation_euler[2] = randrange(6)
#random assign particle hue value
bpy.data.materials["emitter"].node_tree.nodes["Hue Saturation Value"].inputs[0].default_value = random.uniform(0,1)
#random hue value of the background
bpy.data.materials["BG"].node_tree.nodes["Hue Saturation Value"].inputs[0].default_value = random.uniform(0,1)
#show crypto logo if mouth-007 is showing
if bpy.data.objects['Head-002'].modifiers["Mouth-007"].show_render == True:
bpy.data.objects["crypto"].hide_render = False
else:
bpy.data.objects["crypto"].hide_render = True
showhierachy()
ShowInViewport()
#FUNCTION07 - RENDER AND SAVE THE IMAGE
def render(folderPath, filename):
bpy.context.scene.render.filepath = folderPath+"//"+filename+".png"
bpy.ops.render.render(animation=False, write_still=True)
#FUNCTION08 - PUT NUMBER ON THE IMAGE FILE NAME
def batchgenerate(count=5, rarity=4, folderPath="", filename = ""):
for n in range(count):
imageNo = "{0:0=3d}".format(n+1)
generate(rarity)
render(folderPath, filename+imageNo)